Process one measurement
By the end of this chapter, you will have a understanding of how to integrate the Tire Tread SDK into your Android app and provide your users with accurate tire tread depth measurements.
UUID for each tire measurement
This code fragment is used to initialise the Tire Tread SDK for the next tire and retrieve the UUID value for this tire tread measurement.
The first line initialises the Tire Tread SDK for the next tire and stores the result in the 'result' variable. The next step checks if the initialisation was successful by checking the 'getSuccess' method of the 'result' variable. If the initialisation was not successful, the code logs an error message and returns from the method.
The code then tries to retrieve the UUID from the 'result' variable to get the UUID as a string. The UUID is then stored in the “uuid” variable. If there is an error while trying to retrieve the UUID, the code logs an error message.
The UUID must be stored in the app for retrieval of the measurement results and the PDF-report.
// Initialize the TreadSDK and store the result in the 'result' variable ReturnValue returnValue = TreadSDK.getInstance().initTire(); // Check if the initialization was successful if (!returnValue.getSuccess()) { ... } // Try to retrieve the UUID from the 'result' variable AppSettings.uuid = UUID.fromString(returnValue.getValues().getString("uuid")); XLog.i("Scan ID: %s", AppSettings.uuid); ...
Recording View
To set up the RecordingView as the main view of your activity, the code initialises it and uses a “try-catch” block to handle any potential exceptions. Your app must have an activity that serves as the host for the recordingView. The recordingView contains the "Start" and "Stop" buttons and manages their functionality. When the "Start" button is pressed, the transmission of recorded images begins immediately.
try { // This calls the constructor inside the Tread SDK setContentView(R.layout.activity_recording); // get access to the object recordingView = findViewById(R.id.recording_view); } catch (Exception e) { ... }
Receiving Measurement Results with the Tire Tread SDK
Before initialising the Tire Tread SDK, it is important to set the callback for the result handler using the following code:
treadSDKParameters.setCallbackResultHandlerMetric(ResultActivity::resultHandlerMetric);
Once the measurement results are received from the Tire Tread SDK, the resultHandlerMetric()
in the Integrator App will be called.
Here’s an example implementation of the resultHandlerMetric()
:
public static void resultHandlerMetric(ResultMetric result) {
XLog.i("result: Global" + result.regionGlobal + ", regionLeft " + result.regionLeft + ", regionMiddle " + result.regionMiddle + ", regionRight " + result.regionRight);
}
Measurement Sequence Diagram
The Tire Tread SDK measurement process involves calling the following functions in sequence:
initialise the SDK by calling initSDK()
and passing the necessary parameters and callback functions.
Retrieve the tire’s unique identifier by calling initTire()
.
Start image capturing by displaying the RecordingView()
.
Present the measurement results in your app’s user interface by handling either the callbackResultHandlerMetric()
or callbackResultHandlerImperial()
.
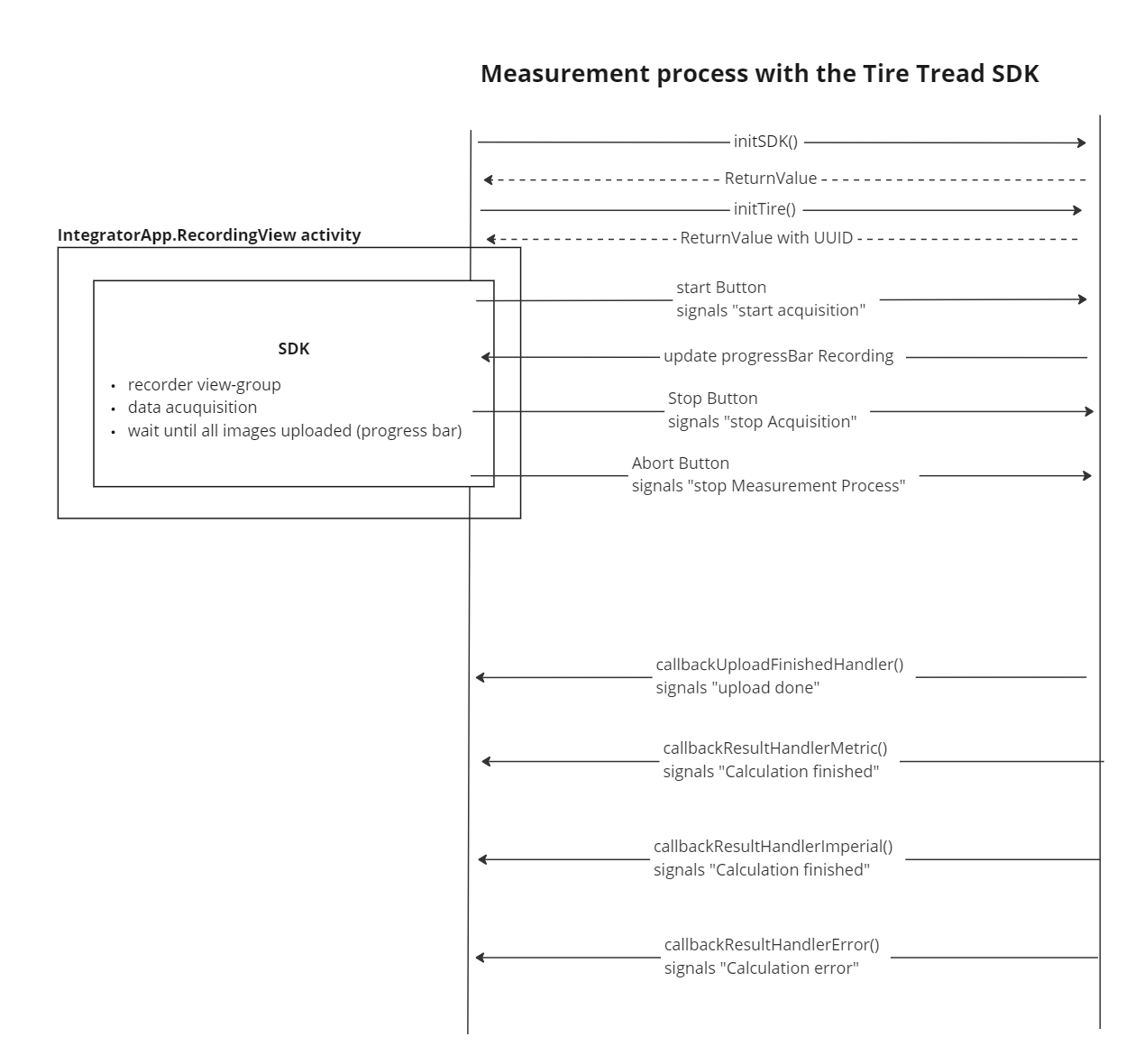
User Corrected Values and Comments
User Comments
To send a comment on a measurement, use the sendComment()
method from the Tire Tread SDK. As usual with the Tire Tread SDK, the UUID for the tire must be passed as a parameter. The comment is passed as a string. The method returns a ReturnValue
object, which can be used to check if the call was successful.
// Get the user's comment String userFeedback = txtUserInput.getText().toString();
// Send the comment to the Tire Tread SDK ReturnValue returnValue = TreadSDK.getInstance().sendComment(AppSettings.uuid, userFeedback);
// Check if the call was successful if (!returnValue.getSuccess()) { ... }
User Corrected Tire Tread Depth Values
To send user corrected tire tread depth values, use the sendManualTreadDepth()
method from the Tire Tread SDK. As usual with the Tire Tread SDK, the UUID for the tire must be passed as a parameter. The measurement system is passed as an eMeasurementSystem
object. The left, middle, and right tread depth values are passed as Double
objects. The method returns a ReturnValue
object, which can be used to check if the call was successful.
returnValue = TreadSDK.getInstance().sendManualTreadDepth(AppSettings.uuid, AppSettings.measurementSystem, left, middle, right);
PDF Report
To retrieve the PDF report for a measurement, use the getReport()
method from the Tire Tread SDK. As usual with the Tire Tread SDK, the UUID for the tire must be passed as a parameter. The method returns a ReturnValue
object, which can be used to check if the call was successful. Additionally is the path to the report stored in the ResultValue when there was a successful call.
Request the report for the tire with the UUID uuid
:
ReturnValue returnValue = TreadSDK.getInstance().getReport(AppSettings.uuid);
Extract the path to the report from the ReturnValue
object:
if (returnValue.getSuccess()) { try {
String filePathToReport = returnValue.getValues().getString("report"); XLog.i("Report path: %s", filePathToReport); ...
}
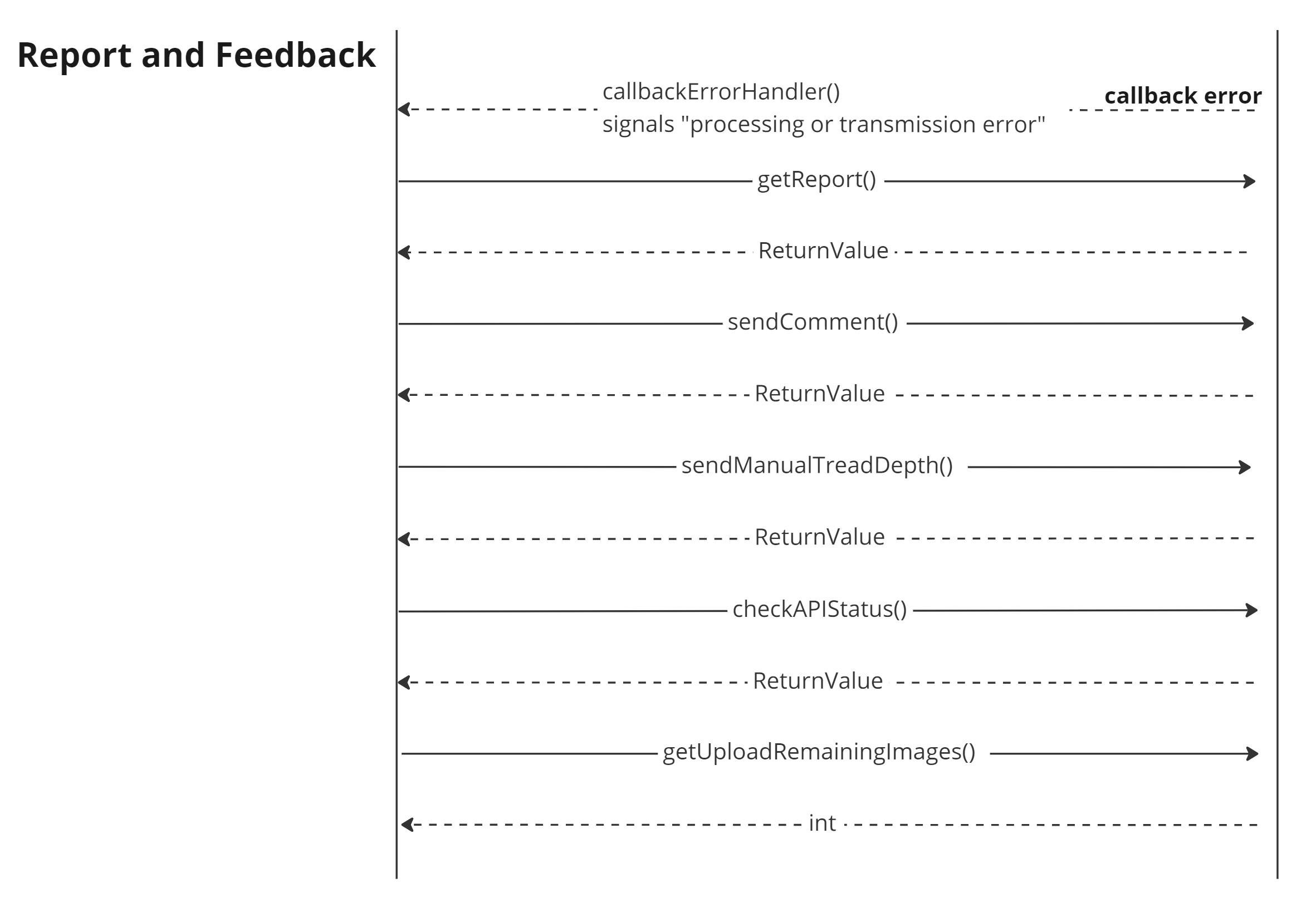
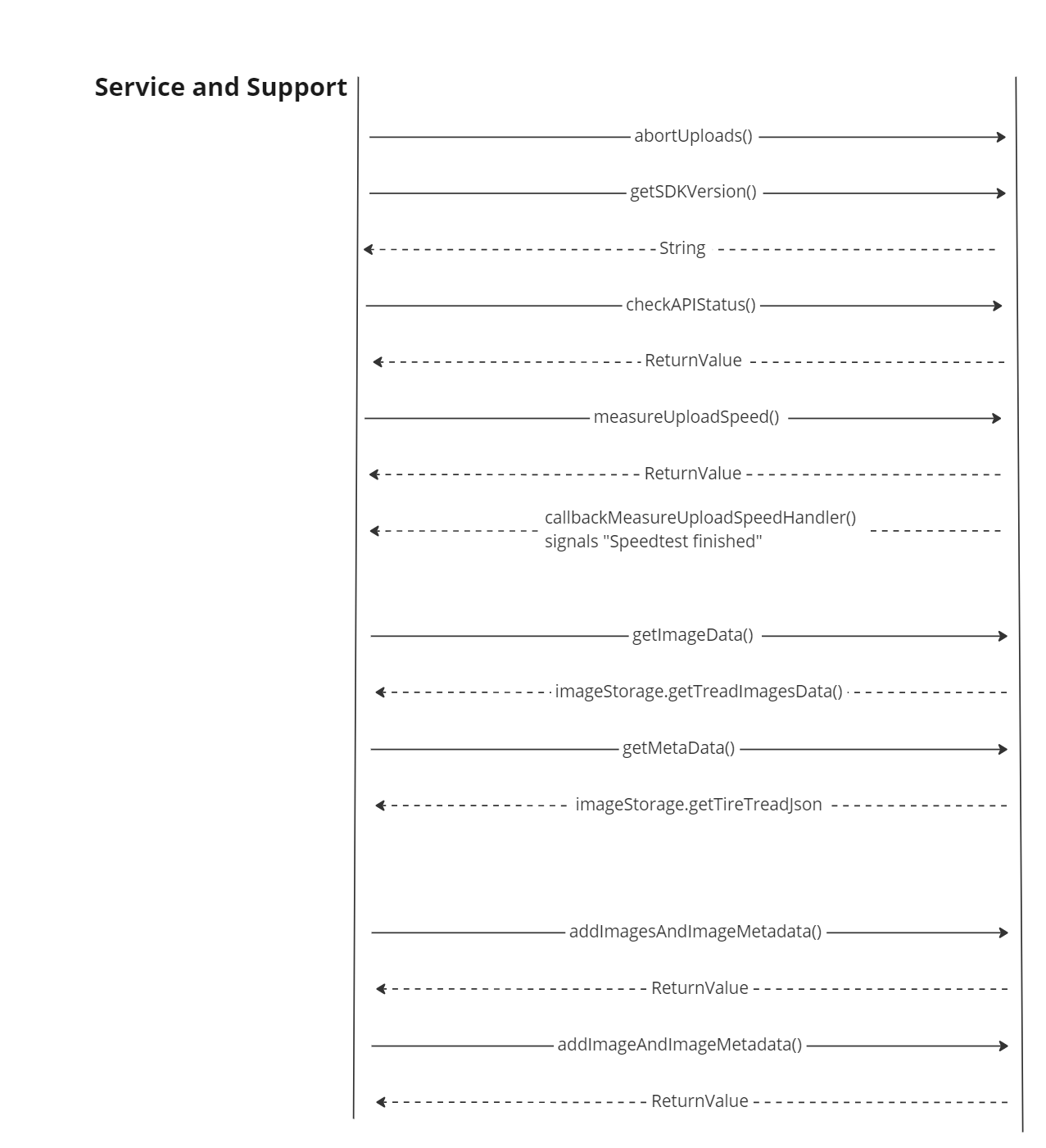